在SwiftUI中,有一個方便的內建修飾器叫做cornerRadius
,它可以讓你輕鬆為視圖設定圓角。通過將cornerRadius
修飾器應用於Rectangle
視圖,你可以將它轉換為圓角矩形。你提供給修飾器的值決定了圓角化效果的程度。
Rectangle()
.cornerRadius(10.0)
另外,在SwiftUI中還提供了一個標準的RoundedRectangle
視圖,用於創建圓角矩形:
RoundedRectangle(cornerRadius: 25.0)
不幸的是,無論是cornerRadius
修飾器還是RoundedRectangle
視圖,都只能對視圖的所有角使用相同的圓角半徑。
如果你需要為視圖的某一角設置圓角呢?
在iOS 17中,SwiftUI框架引入了一個名為UnevenRoundedRectangle
的新視圖。這個視圖的獨特之處在於能夠為每個角指定不同的半徑值,讓開發者可以創建高度客制化的形狀。
如何使用 UnevenRoundedRectangle
有了 UnevenRoundedRectangle
,你可以輕鬆地創建帶有不同半徑圓角的矩形形狀。要使用 UnevenRoundedRectangle
,只需為每個角指定半徑即可。以下是一個示例:
UnevenRoundedRectangle(cornerRadii: .init(
topLeading: 50.0,
bottomLeading: 10.0,
bottomTrailing: 50.0,
topTrailing: 30.0),
style: .continuous)
.frame(width: 300, height: 100)
.foregroundStyle(.indigo)
此外,你還可以指定角的樣式。如指定 continuous
角樣式,就可以使角落看起來更加平滑。如果你將以上程式碼放在 Xcode 15 中,你可以創建以下類似的矩形形狀。
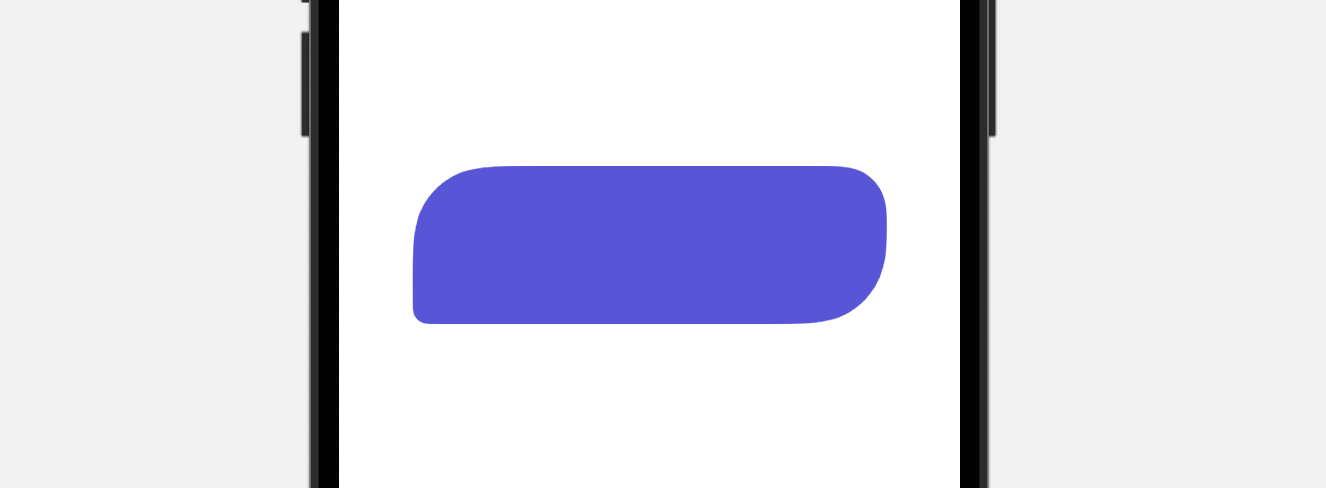
你可以使用這個形狀並通過使用 background
修飾器將其轉換為按鈕。以下是一段示例程式碼:
Button(action: {
}) {
Text("Register")
.font(.title)
}
.tint(.white)
.frame(width: 300, height: 100)
.background {
UnevenRoundedRectangle(cornerRadii: .init(
topLeading: 50.0,
bottomLeading: 10.0,
bottomTrailing: 50.0,
topTrailing: 30.0),
style: .continuous)
.foregroundStyle(.indigo)
}
圓角動畫化
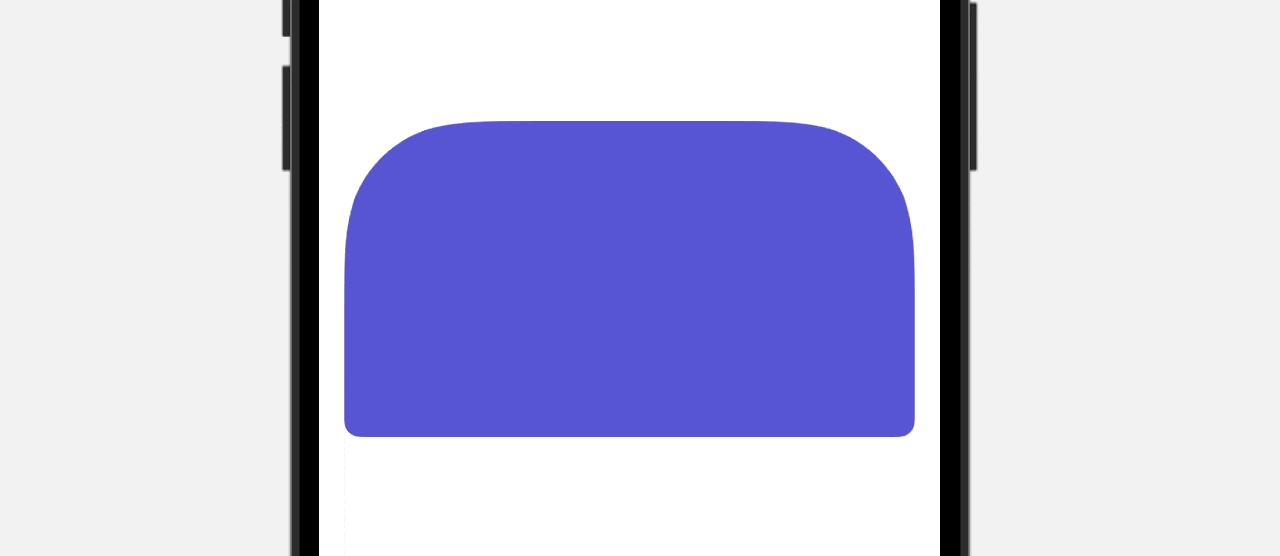
要為 UnevenRoundedRectangle
的圓角添加動畫效果,你可以使用 withAnimation
函式並切換一個Bool
變數。以下是一個範例程式碼片段:
struct AnimatedCornerView: View {
@State private var animate = false
var body: some View {
UnevenRoundedRectangle(cornerRadii: .init(
topLeading: animate ? 10.0 : 80.0,
bottomLeading: animate ? 80.0 : 10.0,
bottomTrailing: animate ? 80.0 : 10.0,
topTrailing: animate ? 10.0 : 80.0))
.foregroundStyle(.indigo)
.frame(height: 200)
.padding()
.onTapGesture {
withAnimation {
animate.toggle()
}
}
}
}
在這個範例中,點擊矩形將切換 animate
變數,該變數控制矩形的圓角半徑。withAnimation
函式將使兩組圓角半徑之間的過渡產生動畫效果。
創建獨特的形狀
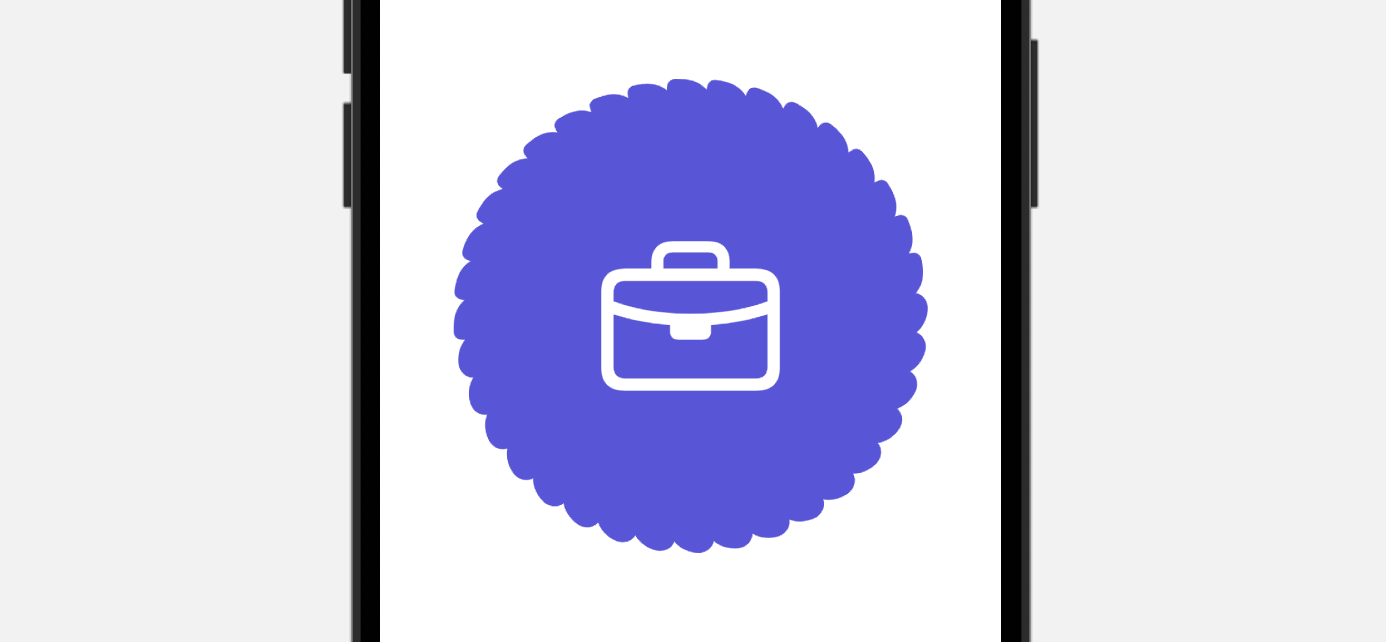
通過重疊多個 UnevenRoundedRectangle
視圖,你可以創造出各種各樣的形狀。上圖的示例就是透過使用以下程式碼做出來的特定形狀:
ZStack {
ForEach(0..<18, id: \.self) { index in
UnevenRoundedRectangle(cornerRadii: .init(topLeading: 20.0, bottomLeading: 5.0, bottomTrailing: 20.0, topTrailing: 10.0), style: .continuous)
.foregroundStyle(.indigo)
.frame(width: 300, height: 30)
.rotationEffect(.degrees(Double(10 * index)))
}
}
.overlay {
Image(systemName: "briefcase")
.foregroundStyle(.white)
.font(.system(size: 100))
}
如要添加額外的視覺效果,你可以通過改變 opacity
的值來產生動畫效果,如下所示:
ZStack {
ForEach(0..<18, id: \.self) { index in
UnevenRoundedRectangle(cornerRadii: .init(topLeading: 20.0, bottomLeading: 5.0, bottomTrailing: 20.0, topTrailing: 10.0), style: .continuous)
.foregroundStyle(.indigo)
.frame(width: 300, height: 30)
.opacity(animate ? 0.6 : 1.0)
.rotationEffect(.degrees(Double(10 * index)))
.animation(.easeInOut.delay(Double(index) * 0.02), value: animate)
}
}
.overlay {
Image(systemName: "briefcase")
.foregroundStyle(.white)
.font(.system(size: 100))
}
.onTapGesture {
animate.toggle()
}
以上這個修改將產生一個非常吸引的視覺效果,可以提升 App 的用戶體驗。
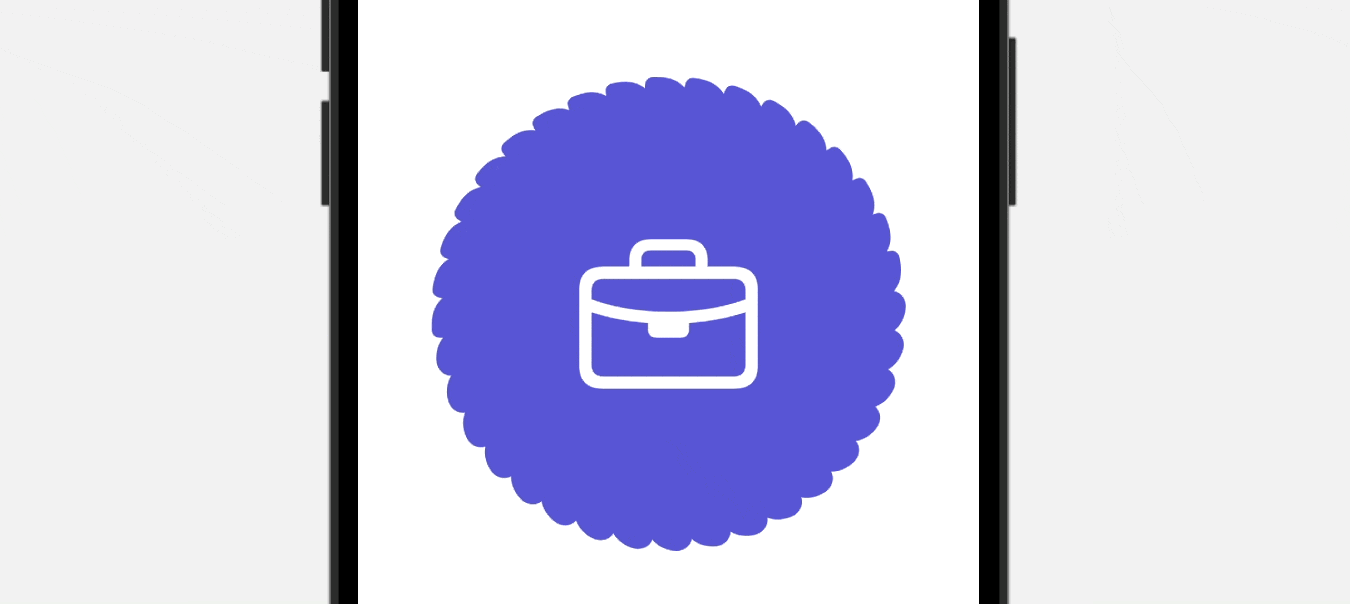
總結
新增的 UnevenRoundedRectangle
視圖為開發者提供了一個方便的解決方案,現在可輕鬆對矩形視圖的特定角落進行圓角處理。你還可以透過 UnevenRoundedRectangle
實作不同的形狀,以增強 App 整體設計和用戶體驗。